Autocomplete with trie (3 solutions) – Code
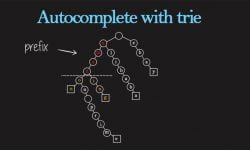
Autocomplete is a feature that search box returns the suggestions based on what you have typed. Autocomplete with trie provides an implementation of auto-complete by using data structure trie. A trie is a tree-like data structure in which every node stores a character. After building the trie, strings or substrings …