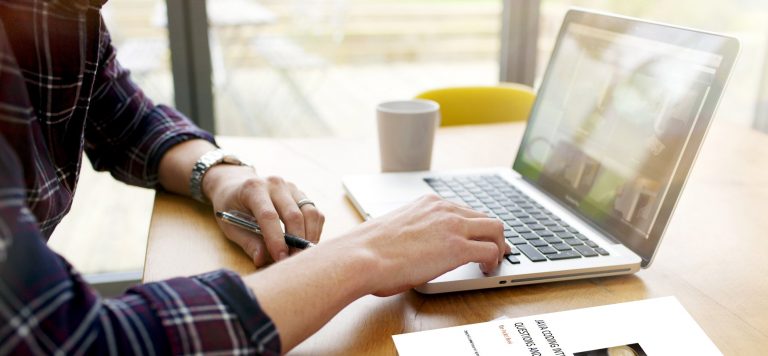
Java coding questions and answers pdf (Free download included)
Table of Content
- List of Java coding questions
- The implementation of data structures
- Java Collections APIs
- Java coding questions and answers PDF
- The format of the PDF
- Source code
List of Java coding questions
Part I Data Structures and APIs
1 Arrays
2 Strings
3 Linked Lists
4 Binary Trees
5 Hashing and Priority Queues
6 Stacks and Queues
7 Graphs
8 Matrices
9 Tries and Nary Trees
10 Intervals and Points
Part II Algorithms and Designs
11 Sorting and Binary Search
12 Recursion
13 Dynamic Programming
14 Numbers and Bits
15 Files, Streams, and Iterators
16 System Designs
Part I Data Structures and APIs
1 Arrays
1.1 Find max
1.2 Find min and max in one pass
1.3 Find top two in one pass
1.4 Find majority elements
1.5 Move all zeros to end
1.6 Find duplicate and missing
1.7 Find duplicate number
1.8 Remove duplicates in place
1.9 Find intersection of two sorted arrays
1.10 Merge two sorted arrays
1.11 Reverse array
1.12 Rotate array
1.13 Next permutation
1.14 Two sum
1.15 Three sum
1.16 Subarray sum to K
1.17 Max sum of subarray
1.18 Max sum of subarray size K
1.19 Max product of subarray
1.20 Self exclude product
1.21 Max profit selling stocks
1.22 Jump game
1.23 Task scheduler
1.24 Trap rain water
1.25 Trap most water
1.26 Max rectangle in histogram
2 Strings
2.1 Reverse string
2.2 Reverse words
2.3 Rotate string
2.4 Find first unique character
2.5 Find repeated substring size K
2.6 Find all subset words
2.7 Ransom note
2.8 Run length encoding
2.9 Swap even odd
2.10 Is anagram
2.11 Find anagram substring indices
2.12 Group anagrams
2.13 Is isomorphic
2.14 Is palindrome
2.15 Longest palindromic substring
2.16 Longest substring with unique chars
2.17 Longest common prefix
2.18 Min window substring
2.19 Min insertions to form palindrome
2.20 Shortest subarray contains all words
2.21 Word wrap
2.22 Word break
2.23 Word ladder
3 Linked Lists
3.1 Find node
3.2 Detect loop
3.3 Delete node
3.4 Delete every Kth node in DLL
3.5 Last men standing
3.6 Print from end
3.7 Reverse linked list
3.8 Reverse K nodes
3.9 Is palindrome
3.10 Find intersection of two lists
3.11 Merge two sorted lists
3.12 Merge K sorted lists
3.13 Sort linked list
3.14 Interleave list
3.15 Flatten doubly linked list
3.16 Add two numbers
3.17 Deep copy random pointers
4 Binary Trees
4.1 Get height
4.2 Get diameter
4.3 Is balanced
4.4 Is BST
4.5 Is complete
4.6 Is subtree
4.7 Is symmetric
4.8 Flip tree
4.9 Find lowest common ancestor
4.10 Find distance of two nodes
4.11 Find in-order successor in BST
4.12 Find Kth largest in BST
4.13 Populate next pointers to right nodes
4.14 Binary tree traversal iteratively
4.15 Level order
4.16 Print vertical
4.17 Print side view
4.18 Print leaves
4.19 Print all root to leaf paths
4.20 Path sum to K
4.21 Max path sum
4.22 Sum of nodes
4.23 Convert sorted array to BST
4.24 Convert sorted linked list to BST
4.25 Convert Binary tree to doubly linked list
4.26 Serialize and deserialize
5 Hashing and Priority Queues
5.1 Object as key
5.2 Remove duplicate contacts
5.3 Get all element counts
5.4 Sort pairs
5.5 Find most popular cost books
5.6 Find year with most population
5.7 Find X to make array sum to K
5.8 Find Kth largest
5.9 Find top K frequent elements
5.10 Find K closest to center
5.11 Find top K tweet words in last hour
6 Stacks and Queues
6.1 Implement stack with queues
6.2 Implement stack with min()
6.3 Validate parentheses
6.4 Infix to postfix
6.5 Prefix to postfix
6.6 Calculate postfix
6.7 Calculate infix
6.8 Implement queue with stacks
6.9 Implement queue with max()
6.10 Remove invalid parentheses
6.11 Find max in sliding window
6.12 Find moving average in sliding window
7 Graphs
7.1 Graph traversal
7.2 Clone graph
7.3 Detect cycle
7.4 Find degree of vertex
7.5 Is bipartite
7.6 Has path from source to destination
7.7 Print all paths from sources to destination
7.8 Find shortest path with BFS
7.9 Find shortest path with Dijkstra’s
7.10 Topological sort with DFS
7.11 Courses order
8 Matrices
8.1 Search in sorted matrix
8.2 Rotate matrix by 90 degrees
8.3 Print matrix in spiral Order
8.4 Fill rows and columns with 1s
8.5 Min distance of meeting point
8.6 Number of islands
8.7 Find path between cells
8.8 Word search on board
8.9 Max region
8.10 Longest increasing path
8.11 Find shortest path between cells
8.12 Nearest bikes
9 Tries and Nary Trees
9.1 Implement trie methods
9.2 Autocomplete
9.3 Find distinct palindromic substrings
9.4 Longest repeated substring
9.5 Get height
9.6 Get diameter
9.7 Find lowest common ancestor
9.8 Find distance of two nodes
9.9 Nary tree traversal iteratively
9.10 Level order
9.11 Build hierarchy tree
9.12 Monarchy succession order
10 Intervals and Points
10.1 Merge intervals
10.2 Insert interval
10.3 Count intervals range
10.4 Schedule meetings
10.5 Weighted job scheduler
10.6 Is overlapped
10.7 Is square
10.8 Draw skyline
10.9 Distance of nearest stores
Part II Algorithms and Applications
11 Sorting and Binary Search
11.1 Bubble sort
11.2 Selection sort
11.3 Insertion sort
11.4 Merge sort
11.5 Quick sort
11.6 Find Kth largest and smallest
11.7 Sort squares
11.8 Binary search in sorted array
11.9 Binary search in rotated sorted array
11.10 Find occurrences in sorted array
11.11 Find peak element
11.12 Find first larger than K
11.13 Find largest smaller than K
11.14 Find missing in consecutive numbers
11.15 Find missing in arithmetic progression
12 Recursion
12.1 Permutation of array
12.2 Permutation of string
12.3 Generate binary strings
12.4 Generate valid parentheses
12.5 Generate strobogrammatic numbers
12.6 Permutation of multiple arrays
12.7 All subsets of an array
12.8 Combination of numbers
12.9 Combination of subset strings
12.10 Combination sum to K
12.11 Nested integers depth sum
12.12 Letter combinations of phone keypad
12.13 Partition numbers to form IP addresses
12.14 Decode numbers
12.15 Operator combinations results
13 Dynamic Programming
13.1 Climb stairs
13.2 Coin change
13.3 Number of ways decoding base 26
13.4 Least square numbers sum to K
13.5 Knapsack
13.6 Edit distance
13.7 Number of distinct subsequence
13.8 Longest increasing subsequence
13.9 Longest common subsequence
13.10 Longest palindromic subsequence
13.11 Number of unique paths in matrix
13.12 Min path sum in matrix
13.13 Max square area
13.14 Range sum query in matrix
13.15 Max rectangle sum in matrix
14 Numbers and Bits
14.1 Prime number
14.2 Random number
14.3 Random number not in array
14.4 Factorial number
14.5 Fibonacci number
14.6 Fizzbuzz
14.7 Power two
14.8 Power X of Y
14.9 Log two
14.10 Square root
14.11 Is binary
14.12 Is odd number
14.13 Reverse number
14.14 Sum of digits
14.15 Is palindromic number
14.16 Is strobogrammatic number
14.17 Add binary
14.18 Add two big string numbers
14.19 Multiply string numbers
14.20 convert string to integer
14.21 Convert decimal to base N
14.22 Base 26 encoding
14.23 Convert integer to roman numeral
14.24 Count set bits in number
14.25 Hamming distance
14.26 Find missing number
14.27 Find single number
15 Files, Streams, and Iterators
15.1 Count words in file
15.2 Reverse content of file in place
15.3 Clean directories recursively
15.4 Find files size larger than 5M
15.5 Add spaces around parentheses in code
15.6 Find Kth largest in stream
15.7 Find median in stream
15.8 Merge K sorted streams in one
15.9 Check palindrome in stream
15.10 Binary tree in-order iterator
15.11 File line iterator
15.12 Rate limiter iterator
16 System Designs
16.1 Dictionary and spell checker
16.2 Phone directory
16.3 Log analyzer
16.4 Tiny url
16.5 Version control snapshot
16.6 Load balancer
16.7 LRU cache
The implementation of data structures
Arrays
Array implementation
Sorted array implementation
Linked Lists
Singly linked list implementation
Doubly linked list implementation
Circular linked list implementation
Binary Trees
Binary tree implementation
Binary search tree implementation
Hashing and Priority Queues
Hash function and hash table implementation
Priority queue implementation with array
Stacks and Queues
Stack implementation with Array
Stack implementation with linked list
Queue implementation with linked list
Circular Queue implementation with array
Graphs
Graph implementation
Tries and Nary Trees
Trie implementation
Suffix trie implementation
Nary tree implementation
Java Collections APIs and their methods
ArrayList APIs
String APIs
Regex APIs
LinkedList APIs
HashMap APIs
HashSet APIs
Hashtable APIs
PriorityQueue APIs
Stack APIs
Queue APIs
Deque APIs
Convert APIs
BigInteger APIs
Date time APIs
Bitwise and Bitshift
File APIs
FileReader and FileWriter APIs
FileInputStream and FileOutputStream APIs
Scanner APIs
Java coding questions and answers PDF
Java coding interview PDF compiles most asked (up to 250) Java coding interview questions and answers. It not only answers the basic must-known questions, such as sorting or file reading, but also includes the hard and even tricky questions from companies such as Google, Amazon.
Java coding questions and answers PDF
$24.95
The format of the PDF
Besides the coverage, the book insight explains how we format the book. The current edition (2nd edition) of the book has PDF and ePub format. The PDF uses “Complete-Code-In-One-Page” format. The question and the code display on one page, not across over to the next page. This format makes it easy to read and compare the similarity between questions. Here are the examples:
The ePub format shows one function per page. It is perfect to be used as a pocket book. You can use it anytime, anywhere on the go.
Source code
The book comes with the source code. The source code provides the full question statement, sample input, and output. It also has an explanation of Big O notations. Sometimes, alternative solutions are available for follow-up questions. Make sure to study them along with the book.